A few months ago, I got an email from a Sweden-based artist and teacher named MC Coble asking if my Morse code laser post could be helpful for an art installation they were working on. MC used Morse code in previous installations like one in Toronto where they sent coded light from a giant dome to people below who then relayed signals with flashlights. As a part of a new project, MC wanted people to be able to enter protest chants into a website and then have a system convert the message to code and flash a light in a gallery window. Did I want to help? You bet I did!
I got super excited about the prospect of helping with this and knew that with a combination of things I’ve used before it would be really doable. The plan was to have a webserver accept messages from a form and transmit them to a Raspberry Pi (cheap mini-computer), which would then flip pins on a relay to blink the light, like this:
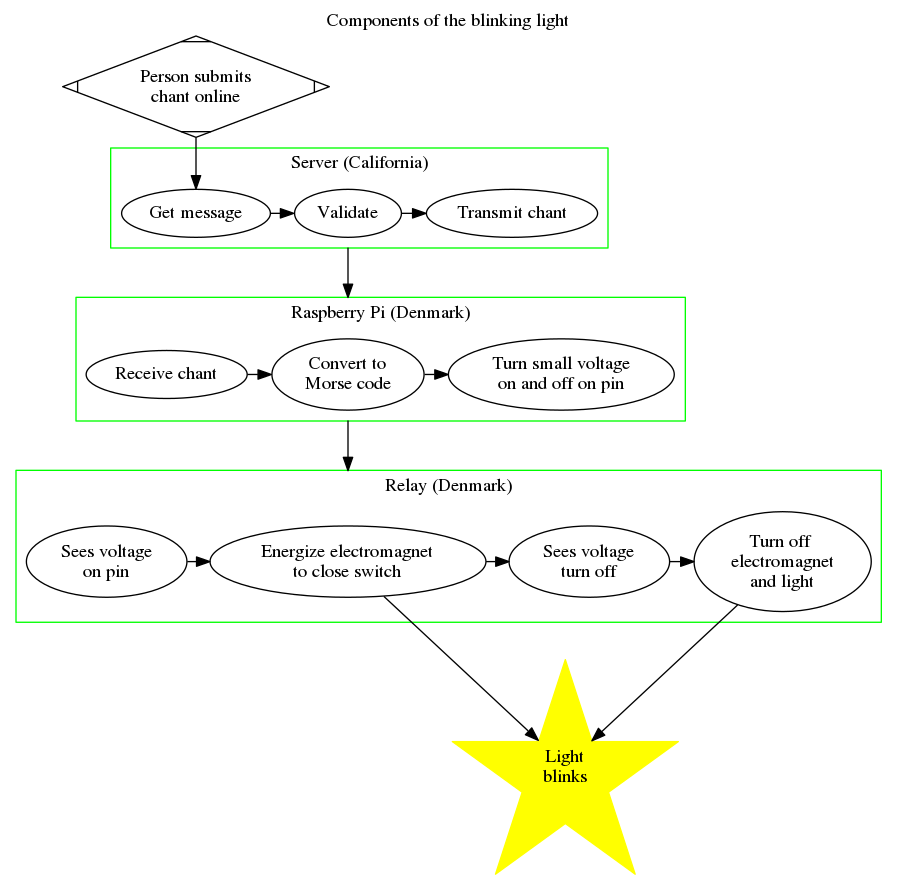
After many emails and some ups and downs, everything worked! This really feels like how the internet is supposed to work.
Continue reading Helping an artist with a Morse code protest chant installation in Denmark